Sooner or later you will need to support Search Engine Optimization in your React app. Fortunately, React uses a virtual DOM and it can easily be rendered on Node.js servers. We just need to generate a Node.js compatible assets bundle, make the server aware of the client side routing and adapt asynchronous data fetching. Let's get started.
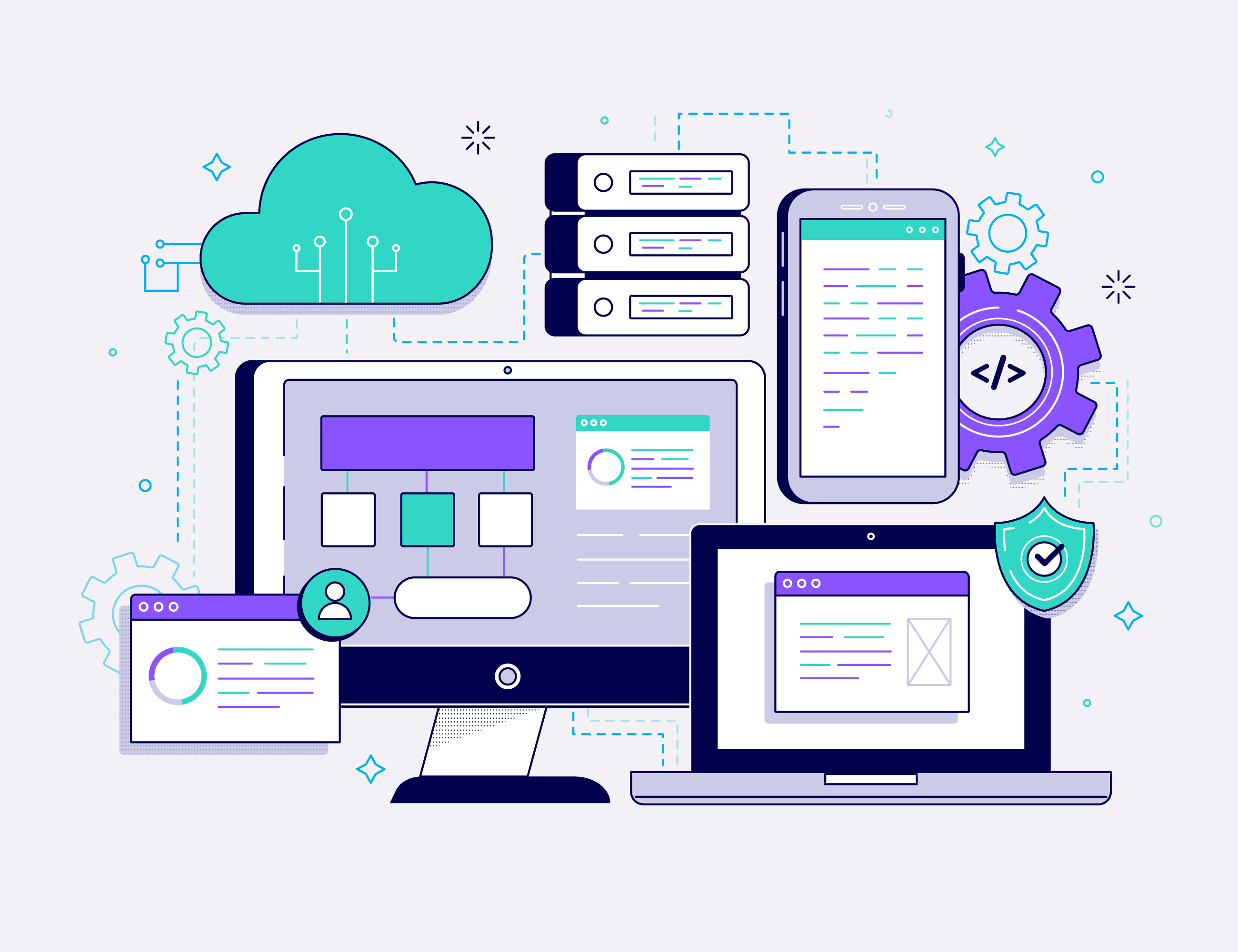
Starting point
We will start with the simplest Typescript stack possible: an express server that serves static files and a "Hello World" React app. We will later be adding client routing and asynchronous data fetching but let's set the basics straight first. Here is the repository structure (more on this git branch).
π client
| π source
| | π app.tsx # React app
| | π index.html
| | π index.tsx # Webpack entry point
| π webpack
| π package.json
| π tsconfig.json
π server
π source
| π server.ts # express static server
π static
π package.json
π tsconfig.json
As per usual, the server returns the static HTML file and the application renders the app on the client side. Let's get the server rendering π οΈ
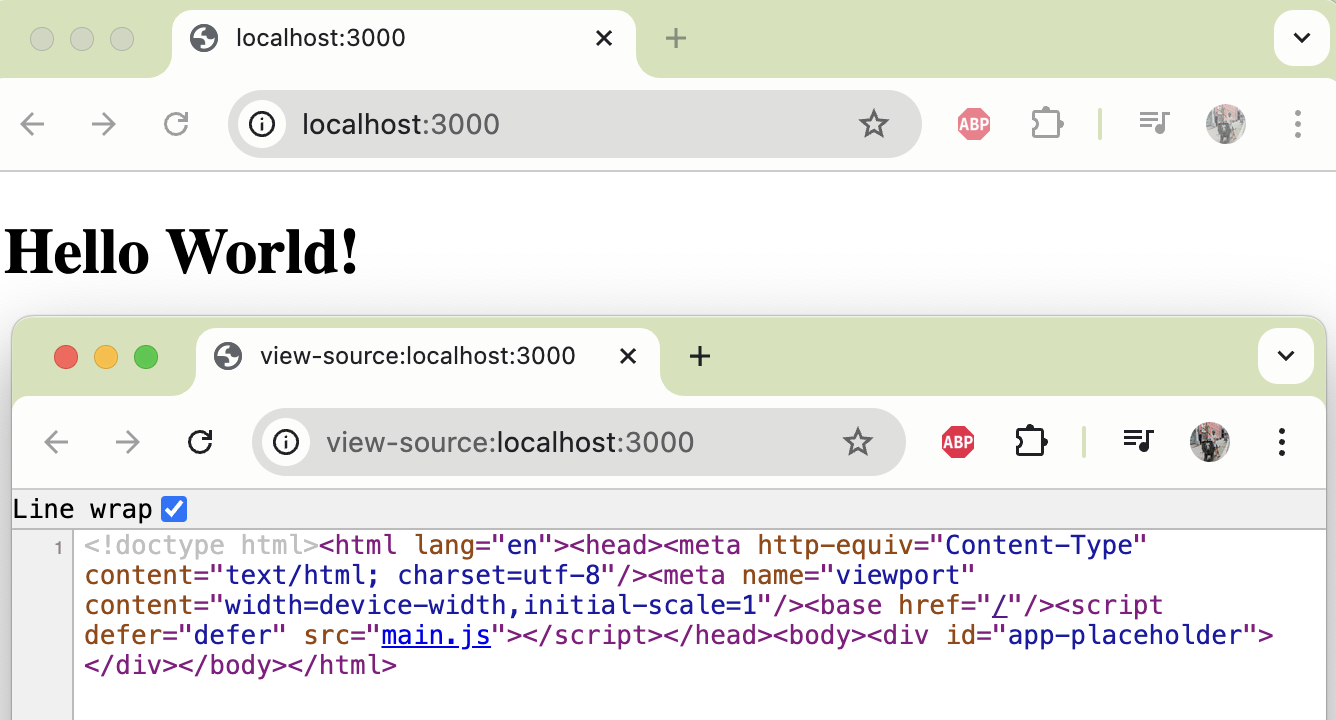
Server SSR setup
First things first: the server can no longer return the static files straightaway. We will need to intercept requests to the index.html file, render the React app on the server (via the renderToString function from react-dom/server) and return the corresponding HTML to the client. There are a few things we need to pay attention to:
- react and react-dom become dependencies of the server app. We will need to install them so Node.js can import them via require.npm i -S react react-dom
npm i -D @types/react @types/react-dom - We need to import the React app from a Node.js compatible bundle. This bundle will be generated from the client project with different Webpack configuration and it needs to be available to the server. In this example, it will be located in a ssr directory (i.e. require('../ssr/app.js')).
- The renderToString function expects a React node, not a React component. We need to wrap the function component in a React.createElement call.
- The renderToString function produces the following HTML, but we need to send a full HTML document to the client. Note that we also need that document to load the client Javascript bundle. A simple way of creating the HTML document is by reading the static file we were previously serving and appending the server rendered HTML into the app-placeholder element.<h1>Hello World!</h1>
Webpack SSR setup
The main issue with the client Webpack bundle is that it is built to run on a browser. Importing it from the Node.js server will raise all sort of errors. On one hand, the client bundle tries to mount the React app to the browser document. Since in Node.js there is no document we will need to skip the mount.
For that we will need a separate webpack file that specifies app.tsx as the entry point and extracts the output to some directory in the server project (in this example, I'm calling the directory ssr). We will also add an npm script to package.json to build the Node.js bundle (i.e. build:ssr).
On the other hand, webpack replaces the export statements and includes the client side dependencies. We need to keep the exports so Node.js can require the React app. And, if some of our dependencies relay on running in the browser, they might raise exceptions when required from the server side. Let's add a couple tweaks to the SSR webpack configuration.
output.libraryTarget / target. Setting this properties to umd and node will make the bundle exports compatible with Node.js.
externals. this configuration option provides a way of excluding dependencies from the output bundles, assuming they will be made available by the consumer of the bundle instead. Used along with webpack-node-externals it will exclude all modules from the node_modules directory.
The generated bundle is now compatible with Node.js, so it can be rendered on the server side π See this change set for more details.
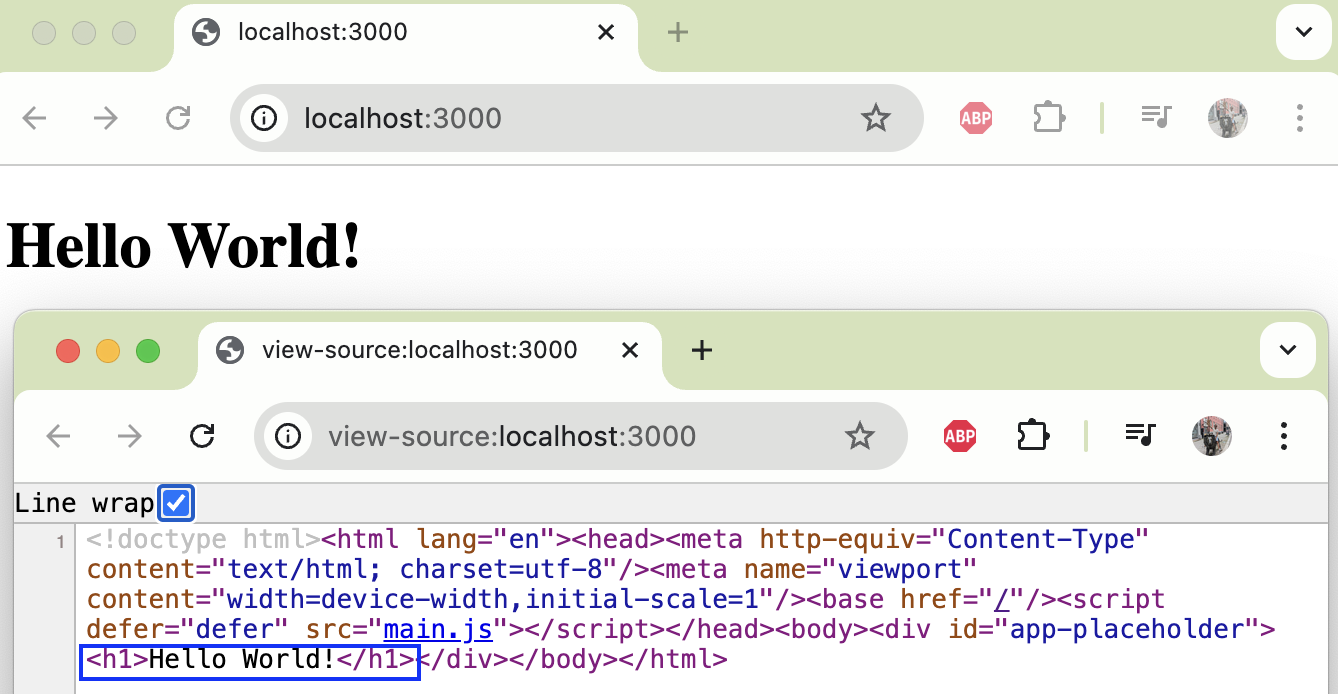
Routing
Most modern apps use client side routing. Let's make our React app a bit more relevant by adding react-router and a couple of different routes. Note that the BrowserRouter must be provided in the index.tsx file; doing so on app.tsx would cause errors on the server side, as the router references the window document.
Now we need to adapt the server rendering to support the in-app routing. We need to install react-router as a dependency of the server and then render the app within a router component. Because BrowserRouter is not supported on the server side, we will use StaticRouter instead. Passing the URL of the http request to the router via the location parameter is enough for it to know which component to render.
Lastly we need to modify the express middleware to intercept requests to the new route (i.e. "/login") and render the app at that path. We now support deep links server rendering π» See this change set for more details .
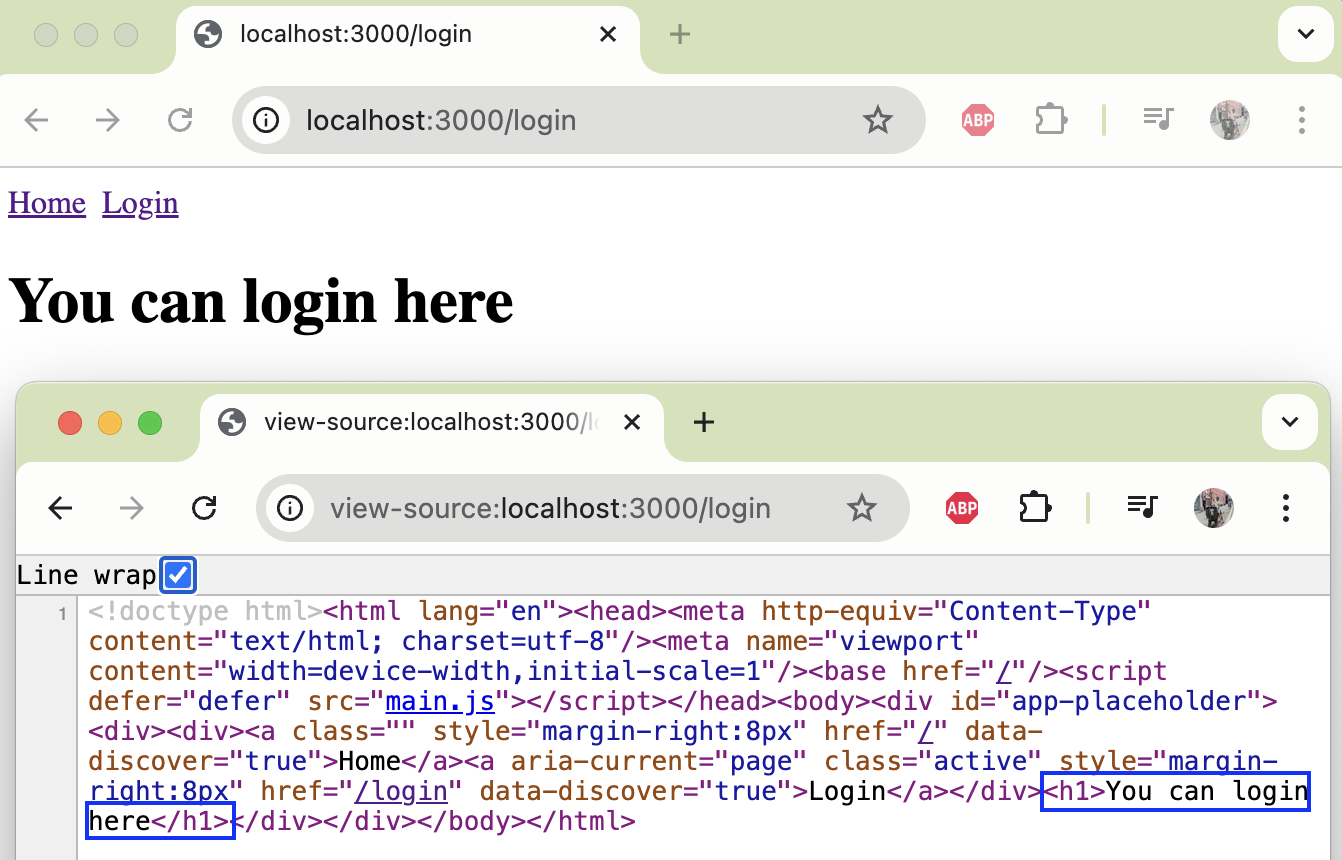
Fetching data on app start
Finally let's fetch some data asynchronously during the app initialization. Let's say we want to fetch a list of user names from the server and display them in the home page (simple use case for demonstration purposes). We will add a new endpoint (e.g. /api/user-names) that retrieves the user names and we will query it during the Home page initialization. In function components this is usually done via useEffect hooks.
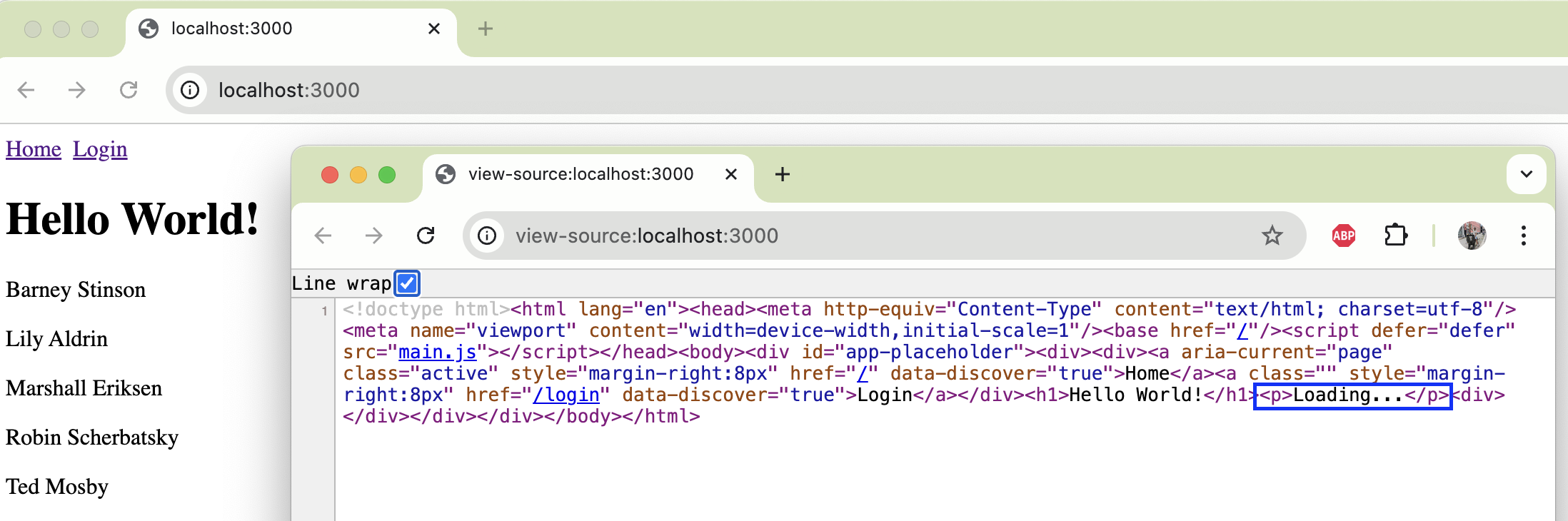
While the client version works, it turns out the server rendered HTML doesn't contain the list of user names. This happens because React doesn't trigger useEffect hooks outside of the browser. Since we want the server rendered HTML to include the list of user names, we will need to fetch them outside of the hooks.
One way to do it is modifying the React app so it can receive the list of user names via props. We can then fetch the list of user names outside of the React lifecycle and render the app once the data is ready, passing the data via props. With this change the resulting server rendered HTML will now contain the list of user names π
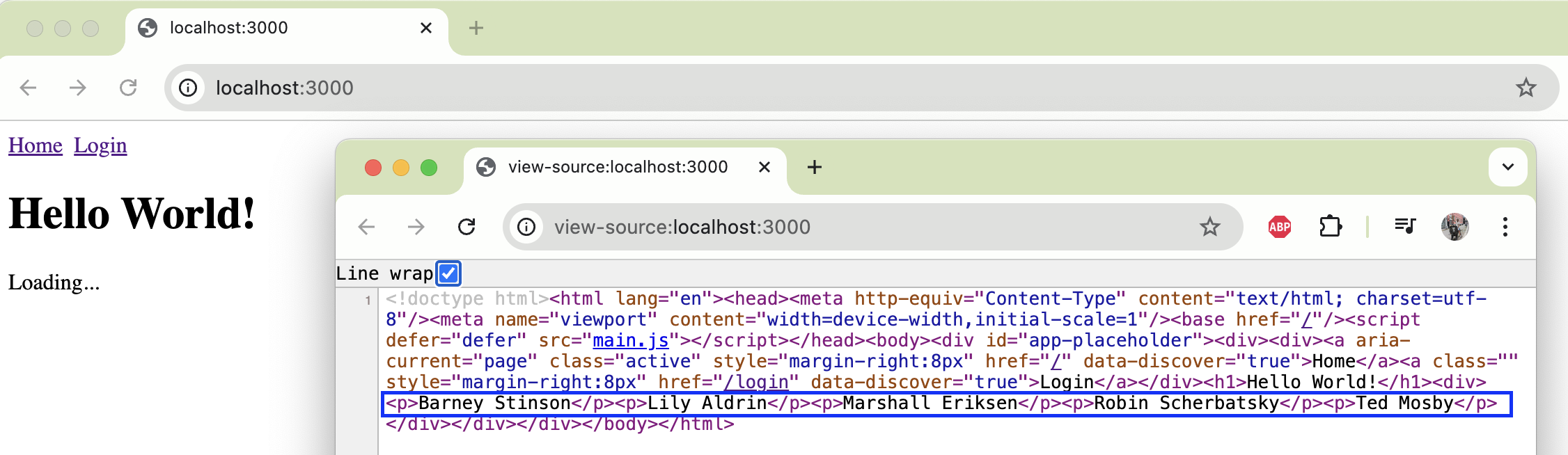
Note however that, when running in the browser, the React app doesn't recognize it had already been rendered in the server; it displays the loader and fetches the list of user names again. If we can prevent the redundant fetch request we will consume less resources and will improve the user experience as well.
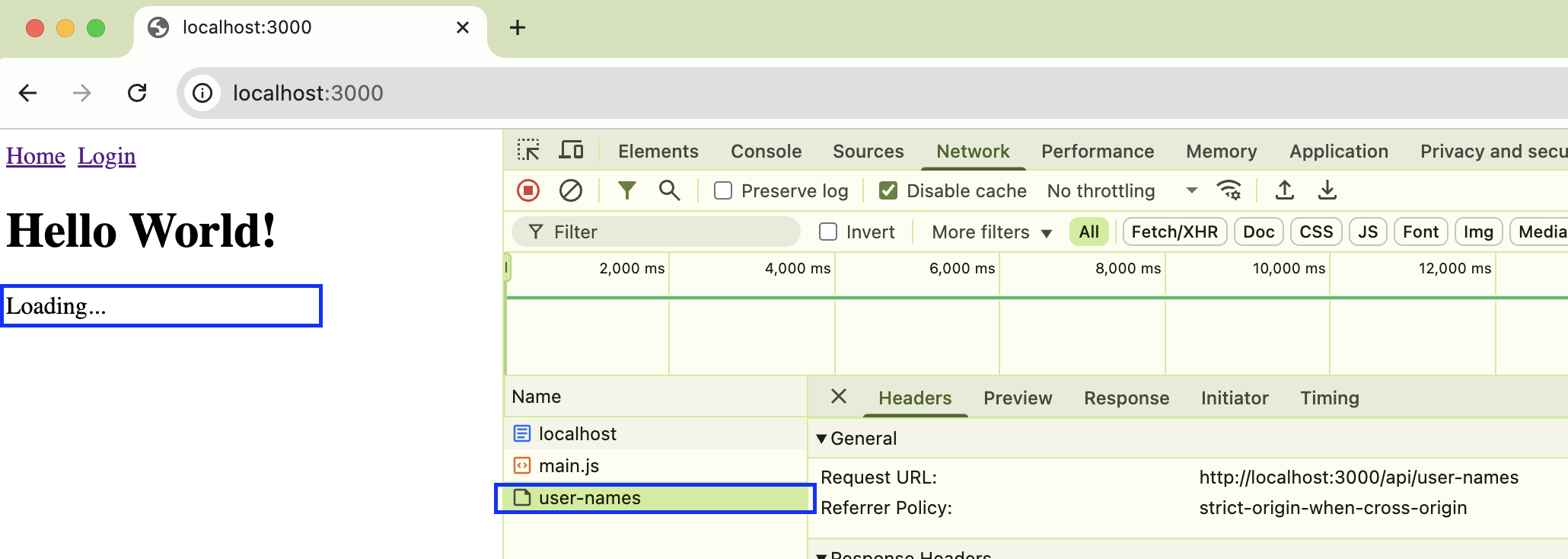
Fortunately it is not complicated. We just need to make the initial state we used on the server side available to the client side, and inject them to React when initializing the app. Note that the useEffect will still run in the browser. We cannot remove it if we want the list to be refreshed upon back and forth navigation, and it is convenient to keep it for development mode as well (the app will not have been rendered in the server). What we can do is preventing the fetch request when we already have a list of user names.
According to the React docs, the hydrateRoot function can be used to βattach React to existing HTML that was already rendered by React in a server environment". At practice though I couldn't observe any different behavior between hydrateRoot(container, <AppWithRouter {...initialState} />) and createRoot(container).render(<AppWithRouter {...initialState} />).
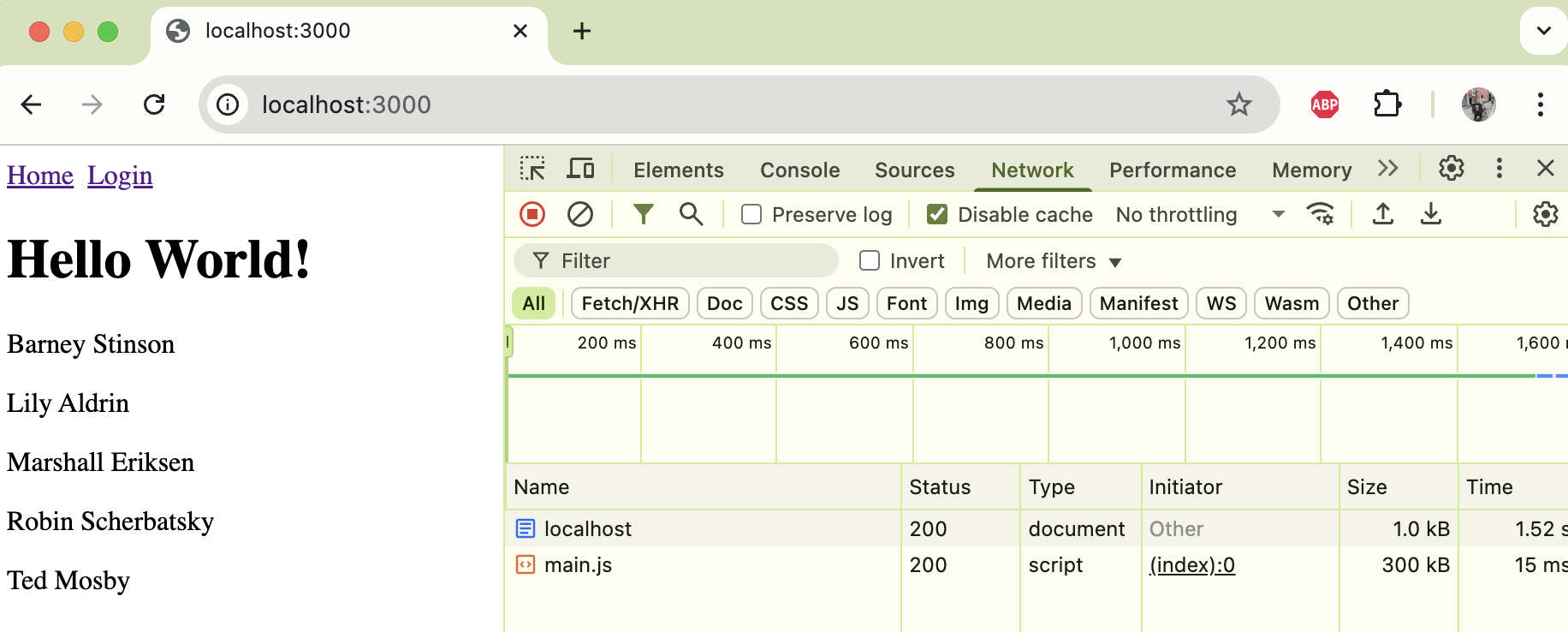
That's it! React SSR with support for deep links and asynchronous data fetching π See this change set for more details and star the entire repository for future reference. Happy coding!
Troubleshooting
A dependency of the client bundle has not been installed on the server (e.g. react, react-dom, react-router, etc.).
Error: Cannot find module 'react'
Require stack:
β- react-ssr/server/source/server.tsThe server assets bundle contains references to document or dependencies that relay on it.
ReferenceError: document is not defined
βat Object.596 (react-ssr/server/static/main.js:2:139274)
βat t (react-ssr/server/static/main.js:2:139761)
βat react-ssr/server/static/main.js:2:139801
βat Object.<anonymous> (react-ssr/server/static/main.js:2:139808)The server assets bundle is not exporting the React app via Node.js exports. Most likely Webpack is not specifying the right target/libraryTarget.
TypeError: App is not a function
βat react-ssr/server/source/server.ts:11:34
βat Generator.next (<anonymous>)
βat react-ssr/server/source/server.ts:8:71renderToString is receiving a React component (i.e. renderToString(App)) instead of a React node (i.e. renderToString(createElement(App))).
Warning: Functions are not valid as a React child. This may happen if you return a Component instead of <Component /> from render. Or maybe you meant to call this function rather than return it.The React app includes a browser based router (e.g. BrowserRouter or HashRouter) which ends up in the server assets. The server version must use a compatible version instead (e.g. StaticRouter).
react-ssr/server/node_modules/react-router/dist/development/index.js:404
let { window: window2 = document.defaultView, v5Compat = false } = options;
ReferenceError: document is not defined
βat getUrlBasedHistory (react-ssr/server/node_modules/react-router/dist/development/index.js:404:27)The server is not providing a router component when rendering the App.
react-ssr/server/node_modules/react-router/dist/development/index.js:336
throw new Error(message);
Error: useLocation() may be used only in the context of a <Router> component.
βat invariant (react-ssr/server/node_modules/react-router/dist/development/index.js:336:11)The server is calling the React app function directly (i.e. renderToString(App)), instead of rendering it within the React lifecycle (i.e. renderToString(createElement(App))).
Warning: Invalid hook call. Hooks can only be called inside of the body of a function component. This could happen for one of the following reasons:
1. You might have mismatching versions of React and the renderer (such as React DOM)
2. You might be breaking the Rules of Hooks
3. You might have more than one copy of React in the same app
See https://reactjs.org/link/invalid-hook-call for tips about how to debug and fix this problem.
react-ssr/server/node_modules/react/cjs/react.development.js:1622
return dispatcher.useState(initialState);
TypeError: Cannot read properties of null (reading 'useState')
βat useState (react-ssr/server/node_modules/react/cjs/react.development.js:1622:21)The server assets bundle includes dependencies that are not supported on the server. Most likely Webpack is not specifying the right externals property.
TypeError: Cannot read properties of null (reading 'useContext')
βat Object.t.useContext (react-ssr/server/ssr/app.js:2:9370)
βat zt (react-ssr/server/ssr/app.js:2:78603)
βat react-ssr/server/ssr/app.js:2:126961
βat renderWithHooks (react-ssr/server/node_modules/react-dom/cjs/react-dom-server-legacy.node.development.js:5662:16)