Perhaps steroids is too much saying, but you can definitely get more out of web development. In this post I will explain some of the techniques we use at Sage to develop our web platforms, both front end and back end, which I've later on exported to my personal projects too. Keep reading to streamline your own development experience!
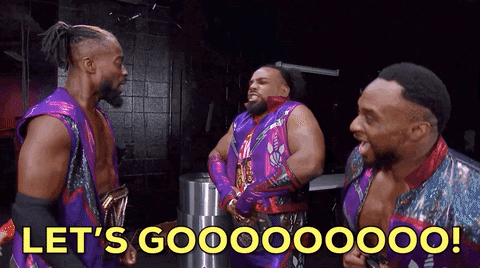
If you are reading this I will understand that you have made your homework and you already have a Git based version control solution, a dependency manager and a module bundler in place. Having the basics covered we can move into more challenging stuff. Be aware that I am using Visual Studio Code, so the solutions I provide are based on that particular IDE. If you prefer other choices, you will have to investigate the available tools out there, but the concepts are still applicable. That being said, let's rock and roll π€©
Type safety
JavaScript was designed in 10 days. There are some things that make it a great language but it also has some flaws: e.g. not being able to detect certain common errors before runtime. Given that you already have to transpile your code, adding a build process to your development pipeline, why not investing a bit more effort and enjoy the safeness only a statically typed language can provide?
Regardless your project size and complexity, having meta data about your code will help it being more descriptive and will allow you to run some compilation checks, using awesome libraries such as Typescript. A very cool aspect of using Typescript with an appropriate IDE is that you get a list of all the attributes and methods available in an object when you type, a feature also known as Intellisense. Once you try it, you will never be able to develop without it again!
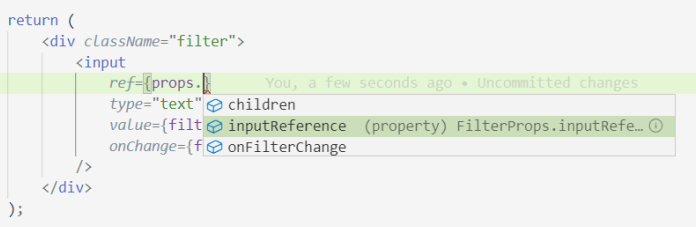
Steps
- Install typescript and a module loader as development dependenciesnpm install --save-dev typescript awesome-typescript-loader
- Add a tsconfig.json configuration file. If you are migrating an existing project to typescript, you will probably want to use the allowJs option to make the transition gradually, as well as waiting until the transition is complete to enable noImplicitAny, strictNullChecks, etc.
- Configure your module bundler to resolve typescript files. In my case I use webpack, so this is how the story goes (webpack.config.js):
- Rename at least your entry point file from *.js to *.ts and surrender to typescript awesomeness
Formatting
Don't lose a single minute more writing semicolons, replacing double quotes with single quotes or arguing with your colleagues about how to format ternary expressions and lines maximum length. Put a formatter that integrates with your IDE in place and it will save you priceless amounts of time while enforcing a standard code guideline.
Prettier is a popular opinionated code formatter that will take care of formatting for you. It allows you to configure a few parameters (e.g. maximum line length) but, as it is opinionated, cannot be very customized (which will save you stupid discussion with your teammates). You can either run it from the command line or use the VSCode extension to automatically format every file each time the file is saved.
Steps
- Install prettier as a development dependencynpm install --save-dev prettier
- Add a prettier.rc json configuration file with your favorite settings or skip this step if you are fine with prettier default settings
- Add npm scripts to run prettier
- Install the VSCode prettier extension (esbenp.prettier-vscode) and add it to .vscode/extensions.json
- Configure VSCode to enable the Format On Save option by adding the following property to .vscode/settings.json. I also recommend you to set the prettier Require Config property to true so prettier formatting will only be applied to projects that contain a prettier configuration file
Linting
Static code analysis that will catch suspicious code (e.g. floating promises) and will enforce the best practices (e.g. functions maximum number of lines). eslint is a great linting tool, which can be highly customized and manages to automatically fix certain types of issues. With a bit of additional configuration it also supports Typescript language, which makes it an ideal candidate to replace the deprecated tslint.
Steps
- Install eslint as a development dependencynpm install --save-dev eslint
- Create a .eslintrc.js configuration file. You can either copy an existing one (remember to install the required dependencies) or create a new one with the eslint command line utilitynpx eslint --init
- Add npm scripts to run eslint
- If you are using or want to use prettier, you will need to configure eslint to respect prettier rulesnpm install --save-dev eslint-config-prettier eslint-plugin-prettier
- Install the VSCode eslint extension (dbaeumer.vscode-eslint) and add it to .vscode/extensions.json
- Configure VSCode to enable the Format On Save option as well as the Format: Enable eslint option by adding the following properties to .vscode/settings.json
- Optionally some more plugins can be installed on top of eslint. For example, I like to enforce the import statements to be alphabetically sorted. We can use eslint-plugin-import for such purposenpm install --save-dev eslint-plugin-import
- Finally, you might need to tweak .eslintrc.js file to meet your needs, specially if you are using Typescript and/or React
Spell checking
So far, words are the most useful concept when it comes to communication between humans. If you want your code to be descriptive, you must avoid variable names such asel,doc,i and use words with actual meaning:element,document,index. And in case you are already paying extreme attention to variable names, even the most literate software engineer introduce typos when they code.
To help us being consistent in the practice of typing meaningful names, we have the amazing Code Spell Checker tool. It will point out invalid words and typos, and it also recognizes the camel case convention so it will understandaNameLikeThis. In addition, it allows defining a white list of words that will not be considered in .vscode/settings.json, for specific software/project words that cannot be found in an english dictionary.
Steps
- Install the VSCode Code Spell Check extension (streetsidesoftware.code-spell-checker) and add it to .vscode/extensions.json
Conclusions
That's it for now! The concepts are not rocket science, but they greatly help me feeling comfortable when working on a project. Bring them in and forget about styling code, writing typos, and searching in the docs for the available members in objects. Discover how to make your development experience even better in the second chapter of this series πΎπ (coming soon to the best theatres). See you in the next post!